KMZ to KML – quick conversion with Python
In this short text, I would like to mention a couple of steps to the successful conversion of the Google Earth files between the .kmz and .kml. As we know, the .kmz file is nothing but a zip-compressed .kml file including map locations viewable in various locations. Sometimes these zipped .kml files are accompanied by some graphic elements, which are used for displaying some additional features. A good example was presented in this article, where I undertook the edition of some internal .kmz file components. In other cases, we might have a few .kml files in the .kmz directory or even just a simple zip-compressed .kml file inside. This text is dedicated to the explanation of how to extract the .kmz file smoothly by Python. I am concentrating, especially on the situation when the .kmz file includes just one .kml object inside. At least in my point of view zipping the .kml file, in this case, is illegitimate.
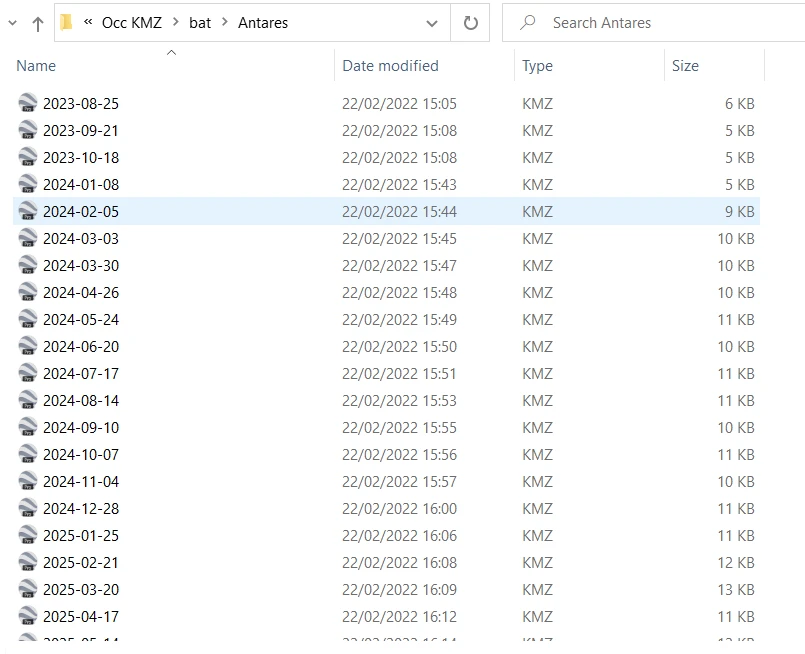
Pic. 1 The list of .kmz files in the folder.
I am starting from the list, which you can see above. There are around 60 .kmz files, which should be .kml initially. In practice, each of these .kmz files stores just one zipped .kml file. This configuration is awkward because the manipulation of the .kmz files is limited in the GIS environment and admittedly the most reasonable application for opening them is Google Earth.
The quickest way can sort everything out for us is using Python. We can split our operation into two steps, the first step will include a simple extract of the stuff from our .kmz files and another step will rename the .kml files in all the folders making their name exactly the same as the initial zipped .kmz directory.
1. Firstly, we should change our file extensions from .kmz to .zip, which should be straightforward in our batch process. For this purpose, just this short Python snippet underneath will be required.
import os, sys for filename in os.listdir(os.path.dirname(os.path.abspath(__file__))): base_file, ext = os.path.splitext(filename) if ext == ".kmz": os.rename(filename, base_file + ".zip")
We should import the os module first, which is responsible for miscellaneous operating system interfaces. Another module to be imported is sys – system-specific parameters and functions. Next, the key method is os.listdir, which allows us to manipulate all the files concerned in the given directory. Another important tool here is the method: os.path.splittext() which lets us divide between the root filename and its extension. The last element is the os.rename() method. The effect is amazing, which can be seen below (Pic. 2).
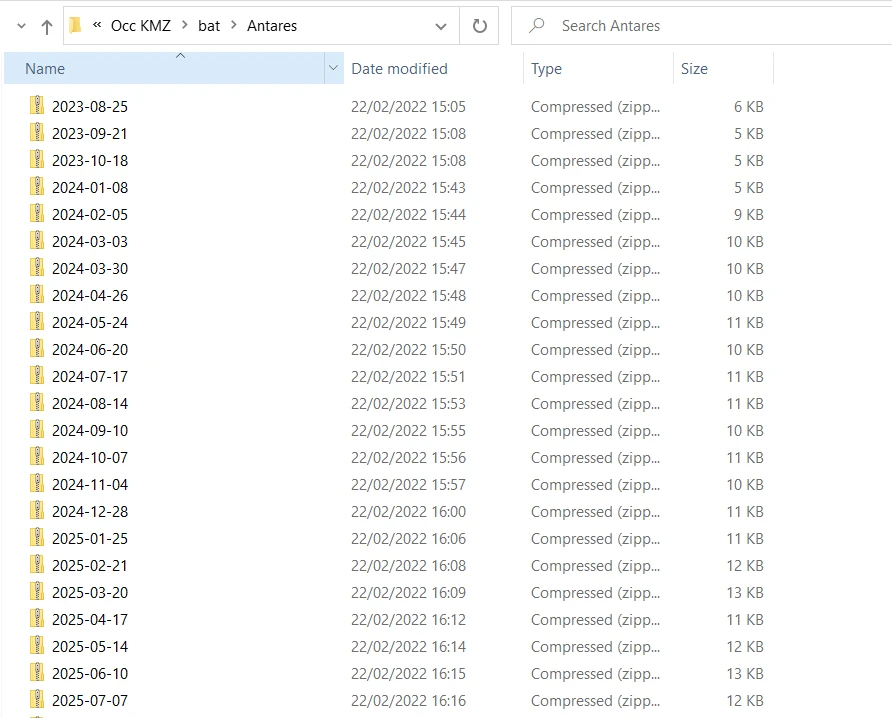
Pic. 2 The effect of batch .kmz files extension changes to .zip
and (apart from obviously Google Earth) now we can see the content inside. We have just one .kml file inside, whose name doesn’t match its mother directory (Pic. 3).

Pic. 3 The content of each .kmz file can now be seen easily since the directory has been converted to .zip.
2. The second step includes batch extraction (unzipping) of all these directories. Occasionally we can quickly sanitize our folder by removing the remaining .zip files.
This snippet of code should extract our files easily.
import zipfile from pathlib import Path p = Path(__file__).parent.absolute() # Extracting all .zip files for f in p.glob('*.zip'): with zipfile.ZipFile(f, 'r') as archive: archive.extractall(path=f'./{f.stem}') print(f'Done {f.stem}')
We have to import the zipfile library this time and pathlib is responsible for giving the correct path for us. Important here will be also the glob function, which helps us to match the specified pattern, which in our case is .zip. In the end, we can extract everything by using the extractall function. The successful result you can see beneath (Pic. 4).
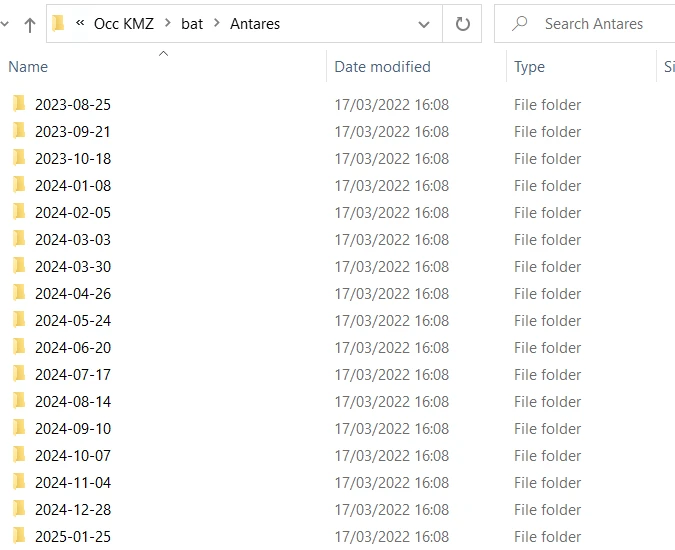
Pic. 4 Unzipped .kml files by Python. Each former .kmz file acts now as the directory.
As it has been told earlier. We can also remove our remaining .zip directories quickly by using the following code below:
import os from pathlib import Path p = Path(__file__).parent.absolute() # Removing all .zip files dir_name = p test = os.listdir(dir_name) for item in test: if item.endswith(".zip"): os.remove(os.path.join(dir_name, item))
Because we should provide the pathlib and os libraries again, both snippets can be merged. It does apply also to the very first code. Therefore I used all of them for creating the KMZ extractor tool, which can be available here.
3. The last step covers bulk changing the name of the .kml files inside the extracted folders. I want them to be called roughly the same as their directories. For this purpose, we can use the last snip of the Python code, which looks as follows.
import os from pathlib import Path path = Path(__file__).parent.absolute() folders = os.listdir(path) for folder in folders: files = os.listdir(r'{}\{}'.format(path, folder)) # Accessing files inside each folder for file in files: # Getting the file extension extension_pos = file.rfind(".") extension = file[extension_pos:] # Renaming the files os.rename(r'{}\{}\{}'.format(path, folder, file), r'{}\{}\{}{}'.format(path, folder, folder, extension))
The pivot method here is the .rfind() method and .rename() method. The first one finds the target files for us and another one renames them.
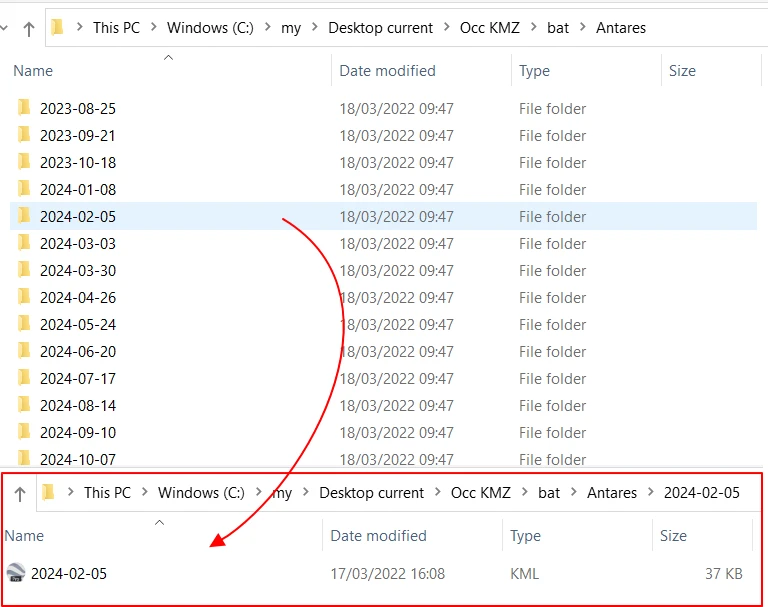
Pic. 5 Renamed .kml files inside the former .kmz directory.
Now, we have our .kml file extracted from the .kmz directory with exactly the same name preserved.
Mariusz Krukar
Links:
- KMZ file extension
- https://fileinfo.com/extension/kmz
- https://www.geeksforgeeks.org/os-module-python-examples/
- https://docs.python.org/3/library/os.html
- https://docs.python.org/3/library/sys.html
- Python os.listdir(path) method
- https://www.geeksforgeeks.org/python-os-path-splitext-method/
- https://www.tutorialspoint.com/python/os_rename.htm
- https://www.geeksforgeeks.org/working-zip-files-python/
- https://docs.python.org/3/library/pathlib.html
- https://docs.python.org/3/library/zipfile.html
- https://www.geeksforgeeks.org/python-string-rfind-method/
- https://www.igismap.com/kmz-to-kml/
Forums:
- https://gis.stackexchange.com/questions/40944/converting-kmz-file-to-kml
- https://stackoverflow.com/questions/16736080/change-the-file-extension-for-files-in-a-folder
- https://stackoverflow.com/questions/5137497/find-the-current-directory-and-files-directory
- https://stackoverflow.com/questions/31346790/unzip-all-zipped-files-in-a-folder-to-that-same-folder-using-python-2-7-5
My questions: